【Three.js】簡単なオブジェクト+パーティクルを表示
2022-08-26
2022-08-26
はじめに
こんにちは!
今回はTree.jsを使用して簡単なオブジェクトとパーティクルを実装してみました。
学習用のお試しなので悪しからず!
実装
今回はこちらのサイトの実装を参考にさせていただきました。
https://www.mitsue.co.jp/knowledge/blog/frontend/201912/20_0000.html
まず、下記からthree.jsをダウンロードします。
https://threejs.org/
同階層に、下記のhtmlファイルを作成します。
index.html
```<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<!-- three.jsを読み込む -->
<script src="three.js"></script>
<!-- index.jsを読み込む -->
<script src="index.js"></script>
</head>
<body>
<section style="z-index: 20; position: absolute">
<canvas id="myCanvas"></canvas>
</section>
</body>
</html>
```
こちらのcanvasタグにスクリーンが描画されます。
そうしましたら、jsファイルを実装していきましょう。
今回は単純な表示なので、initメソッド内に全て記述していきます。
index.js
```window.addEventListener('DOMContentLoaded', init);
function init() {
const width = 1500;
const height = 1200;
// レンダラーを作成
const renderer = new THREE.WebGLRenderer({
canvas: document.querySelector('#myCanvas'),
alpha: true,
});
renderer.setPixelRatio(window.devicePixelRatio);
renderer.setSize(width, height);
renderer.set
// シーンを作成
const scene = new THREE.Scene();
// カメラを作成
const camera = new THREE.PerspectiveCamera(45, width / height, 1, 10000);
camera.position.set(0, 0, +1000);
const hatMaterial = new THREE.MeshLambertMaterial({ color: 0x333333 })
const hat = new THREE.Mesh(
//円柱のジオメトリー(上面半径,下面半径,高さ,円周分割数)
new THREE.CylinderGeometry(25, 25, 40, 30),
hatMaterial
);
hat.position.set(0, 50, 0); //(x,y,z)
const hat_line = new THREE.Mesh(
new THREE.CylinderGeometry(26, 25, 20, 30),
new THREE.MeshLambertMaterial({ color: 0xe60033 })
);
hat_line.position.set(0, 35, 0);
const hat_collar = new THREE.Mesh(
new THREE.CylinderGeometry(40, 40, 5, 30),
hatMaterial
);
hat_collar.position.set(0, 32, 0);
const whole_hat = new THREE.Group();
whole_hat.add(hat, hat_line, hat_collar);
scene.add(whole_hat);
// 平行光源
const light = new THREE.DirectionalLight(0xFFFFFF);
light.intensity = 20; // 光の強さを倍に
light.position.set(1, 1, 1);
// シーンに追加
scene.add(light);
const ambient = new THREE.AmbientLight(0xf8f8ff, 0.9);
scene.add(ambient);
const geom = new THREE.Geometry(50000, 50000, 50000);
//マテリアル(サイズ・頂点色彩を使うか・色)
const material = new THREE.PointsMaterial({
size: 4, //サイズ
transparent: true,// 透過true
opacity: 0.6, //透過性の数値
vertexColors: true, //頂点色彩を使うか
sizeAttenuation: true,//カメラの奥行きの減退
color: 0x3EDBF0 //色
});
//5000個のランダムな頂点作成
const range = 500;
for (var i = 0; i < 5000; i++) {
//THREE.Vector3(x,y,z)
const particle = new THREE.Vector3(
Math.random() * range - range / 2,
Math.random() * range - range / 2,
Math.random() * range - range / 2);
//頂点をジオメトリに追加
geom.vertices.push(particle);
//頂点の色を追加
const color = new THREE.Color(0xeffffc);
geom.colors.push(color);
}
let cloud = new THREE.Points(geom, material);
cloud.name = "particles";
scene.add(cloud);
let zoom = 1000;
// 初回実行
tick();
function tick() {
requestAnimationFrame(tick);
// 箱を回転させる
whole_hat.rotation.y += 0.01;
whole_hat.rotation.x += 0.01;
whole_hat.rotation.z += 0.01;
cloud.rotation.y += 0.005;
cloud.rotation.x += 0.005;
cloud.rotation.z += 0.005;
zoom -= 1;
camera.position.set(0, 0, +zoom);
if(zoom <= 300) {
zoom = 1000;
}
// レンダリング
renderer.render(scene, camera);
}
}
```
こちらで実装は完了です。
それでは、表示してみましょう!
下記のようになりましたでしょうか??
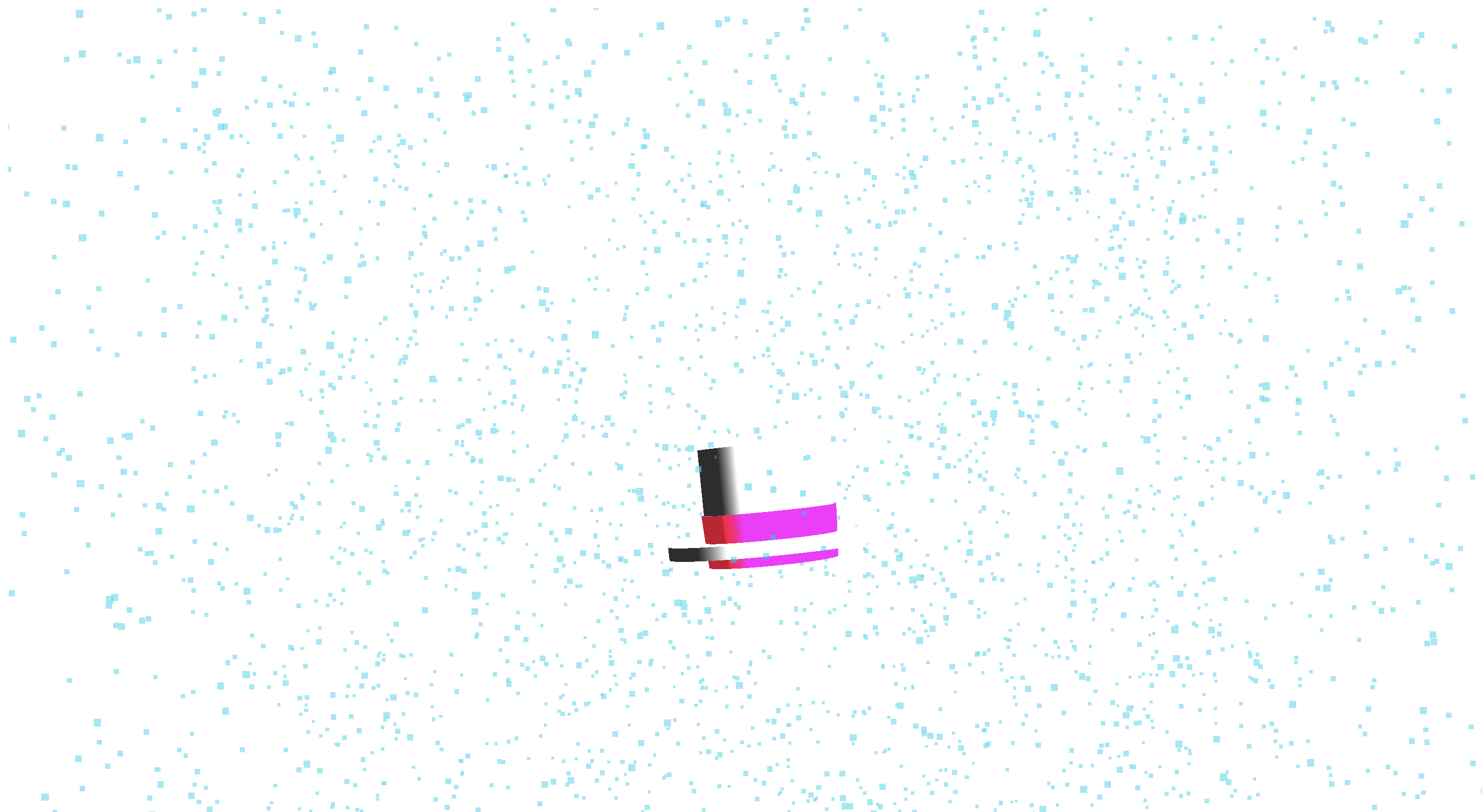
思ったより簡単に3Dオブジェクトが実装できたかと思います。